3 Plots In One Figure Python
It is quite easy to do that in basic python plotting using matplotlib library. We start with the simple one, only one line: import matplotlib.pyplot as plt plt.plot(1,2,3,4) # when you want to give a label plt.xlabel('This is X label') plt.ylabel('This is Y label') plt.show. Apr 28, 2020 Created: April-28, 2020 Updated: December-10, 2020. Use Matplotlib addsubplot in for Loop; Define a Function Based on the Subplots in Matplotlib The core idea for displaying multiple images in a figure is to iterate over the list of axes to plot individual images.
- Python Plot Figure Size
- 3 Plots In One Figure Python Geeks
- Multiple Plots In Python
- Python Plot New Figure
Learning Objectives:
- To be able to produce figures with multiple plot frames, with distinct axes labels and error bars.
- To describe the interactive nature of programming to produce plots
Keywords: figure, axes, frame
In this section, we will discuss, in more detail, how Python produces plots and how we can produce multiple plots within a single programme, within the same figure.
3.3.1 Sizing and positioning plot frames
When a plot is drawn, it is drawn inside an abstract grid with its own coordinates, known as a plot frame . By default, Python chooses the size and positioning of the frame in which a plot is subsequently drawn. In particular, the bottom-left of a plot frame is taken to lie at the origin of this abstract grid, and the height and width of the plot frame are chosen to be something convenient, by default!
You can specify the size and shape of a plot frame by using the function add_axes()
. The arguments of add_axes()
are the x and y coordinates of the bottom-left of the frame, and the width and height of the frame. In particular, if the x and y coordinates of the bottom-left of the plot frame are x
and y
respectively, and the width and height of the plot frame are w
and h
respectively, the syntax to add this frame to a figure is:
plt.figure(1).add_axes((x, y, w, h))
Notice that the arguments of add_axes()
are entries enclosed in curcly brackets ( )
, instead of square brackets [ ]
as for a list. The former object is a new kind of object known as a tuple: essentially a set of entries, seperated by commas, but enclosed in curly brackets ( )
. The following example defines a tuple
For our purposes, you don’t need to know anything else about tuples, just that, in this case, they are passed to the function add_axes()
.
Let’s see how this works by adapting the example from 3.1.3 (a simple scatter plot). In this case we will set the x and y values of the bottom-left of the plot frame to be at the origin and we will set the width and height of the plot frame to be 1.5 and 0.5 respectively:
In this case, we obtain exactly the same plot obtained in example 3.1.3, but now the frame in which the plot is drawn has a size and position that we specified initially.
Exercise 3.3.1
1)
In the following code box, change the values of the entries of the tuple that is passed to add.axes()
in order to generate a plot with various different sizes and positions.
The first two entries of the tuple passed to add_axes()
can be positive or negative (to move the bottom-left corner of the frame up or down as required), but the last two entries, corresponding to the width and height of the plot, must be positive.
3.3.2 Adding and sizing/positioning a new plot frame
You can conveniently add a new plot frame to a figure, in exactly the same way as in the examples shown above, with a new position for the bottom-left of the frame and new width and height. This is how you could, for example, add a plot below (or above, or next to) an existing plot in a figure.

In the following example we will repear the example shown in 3.1.6 (Multiple plots in the same figure) and we will add a second plot frame below the first plot frame, with the same width but whose height is $0.2$ and where the top of the second frame coincices with the bottom of the first frame. We will add the same pair of plots that appear in 3.1.6 to each of the two frames:
Notice that in line-16 above, plt.figure(1)
appears again, which we recall is the syntax for creating figure 1. In this case, we aren’t creating a new figure (figure 1 already existed since line 10), but we’re adding another frame to figure 1.
3.3.3 The “active frame”
Let’s do something odd; let’s take the previous example and move the bit where we added the plot of xvalues
vs other_yvalues
(on line 19) to before the line where we created the second frame (line 16). Can you guess what will happen? Let’s see what happens:
In this case, the code adds both plots (of xvalues
vs yvalues
and xvalues
vs other_yvalues
) to the first frame. This happened because we added the second plot before we created the second plot frame. When we create a plot frame, this frame becomes the active frame : every line of code that follows it applies only to that frame , until we create another frame. In jargon we say that “the plotting environment is interactive”. This essentially means that if write code that applies something to a plot, it applies to the active frame, and to do something to a plot in another plot frame, you have to switch the active frame to the new frame. This is why the line to generate the second plot, in the second frame, had to come after the second plot frame became the active frame.
3.3.4 Removing axes ticks
Let’s consider the following example, where we have placed two identical plot frames side-by-side, using the method described in 3.2.2 . We’ve assumed we have two data sets, ranging over the same y-values, but with two sets of x-values
An immediately unappealing feature of this figure is that the, even though these data are usefully depicted with a shared y-axis, the y-axis values are printed on the left of the right plot frame. In instances like this, you might want to just get rid of the ticks on one of the axes!
You can do this by using a combination of the functions gca()
, get_xaxis()
/get_yaxis()
and set.ticks()
.
The syntax to remove the ticks from either the x or y axis of the plot in the active frame is:
plt.gca().axes.get_xaxis().set_ticks([])
to remove the x-ticks
plt.gca().axes.get_yaxis().set_ticks([])
to remove the y ticks
This is essentially complicated function call, but for now you can see how this works in practice and then you can simply copy and paste this line into your own code.
Going back to the previous example, we wanted to remove the ticks along the y-axis for the plot in the second plot frame. To achieve this we should add the line plt.gca().axes.get_yaxis().set_ticks([])
after the second plot frame has been created. We do this in the following example:
This looks much better!
Exercise 3.3.2
1)
Determine whether it matters if you label the axes of a plot, or change the axes limits, before or after changing the orientation of a plot.
New plotting interface¶
This package provides a simple command line interface to the Mantidplotting functionality, resembling matplotlib and its pyplot package(http://matplotlib.org). The module is subject to changes and feedbackis very much welcome!
New Python command line interface for plotting in Mantid (a la matplotlib)¶
The idea behind this new module is to provide a simpler, morehomogeneous command line interface (CLI) to the Mantid plottingfunctionality. This new interface is meant to resemble matplotlib asfar as possible, and to provide a more manageable, limited number ofplot options.
The module is at a very early stage of development and provideslimited functionality. This is very much work in progress at themoment. The module is subject to changes and feedback is very muchwelcome!
Simple plots can be created and manipulated with a handul ofcommands. See the following examples.
Plot an array (python list)¶
Plot an array with a different style¶
The plot commands that are described here accept a list of options(kwargs) as parameters passed by name. With these options you canmodify plot properties, such as line styles, colors, axis scale,etc. The following example illustrates the use of a few options. Youcan refer to the list of options provided further down in thisdocument. In principle, any combination of options is supported, aslong as it makes sense!
If you have used the traditional Mantid command line interface inPython you will probably remember the plotSpectrum, plotBin and plotMDfunctions. These are supported in this new interface as shown in thefollowing examples.
Plot a Mantid workspace¶
You can pass one or more workspaces to the plot function. By defaultit will plot the spectra of the workspace(s), selecting them by theindices specified in the second argument. This behavior is similar tothe plotSpectrum function of the traditional mantidplot module. This isa simple example that produces plots of spectra:
Different types of plots¶
The plot() function provides a unified interface to different types ofplots, including specific graphs of spectra, bins, multidimensionalworkspaces, etc. These specific types of plots are explained in thenext sections. plot() makes a guess as to what tool to use to plot aworkspace. For example, if you pass an MD workspace it will make an MDplot. But you can request a specific type of plot by specifying akeyword argument (‘tool’). The following tools (or different types ofplots) are supported:
Tool | tool= parameter values (all are equivalent aliases) | Old similar function |
---|---|---|
plot spectra (default) | ‘plot_spectrum’, ‘spectrum’, ‘plot_sp’, ‘sp’ | plotSpectrum |
plot bins | ‘plot_bin’, ‘bin’ | plotBin |
plot MD | ‘plot_md’, ‘md’ | plotMD |
The last column of the table lists the functions that produce similarplots in the traditional MantidPlot Python plotting interface. For thetime being this module only supports these types of specificplots. Note that the traditional plotting interface of MantidPlotprovides support for many more specific types of plots. These orsimilar ones will be added in this module in future releases:
- plot2D
- plot3D
- plotSlice
- instrumentWindow
- waterFallPlot
- mergePlots
- stemPlot
Plot spectra using workspace objects and workspace names¶
It is also possible to pass workspace names to plot, as in thefollowing example where we plot a few spectra:
Let’s load one more workspace so we can see some examples with list ofworkspaces
The next lines are all equivalent, you can use workspace objects ornames in the list passed to plot:
Here, the plot function is making a guess and plotting the spectra ofthese workspaces (instead of the bins or anything else). You can makethat choice more explicit by specifying the ‘tool’ argument. In thiscase we use ‘plot_spectrum’ (which also has shorter aliases:‘spectrum’, or simply ‘sp’ as listed in the table above):
Alternatively, you can use the plot_spectrum command, which isequivalent to the plot command with the keyword argumenttool=’plot_spectrum’:
Plotting bins¶
To plot workspace bins you can use the keyword ‘tool’ with the value‘plot_bin’ (or equivalent ‘bin’), like this:
or, alternatively, you can use the plot_bin command:
Plotting MD workspaces¶
Similarly, to plot MD workspaces you can use the keyword ‘tool’ withthe value ‘plot_md’ (or ‘md’ as a short alias), like this:
or a specific plot_md command:
For simplicity, these examples use a dummy MD workspace. Please referto the Mantid (http://www.mantidproject.org/MBC_MDWorkspaces) for amore real example, which necessarily gets more complicated and dataintensive.
Changing style properties¶
You can modify the style of your plots. For example like this (for afull list of options currently supported, see below).
Notice that the plot function returns a list of lines, whichcorrespond to the spectra lines. At present the lines have limitedfunctionality. Essentially, the data underlying these lines can beretrieved as follows:
If you use plot_spectrum, the number of elements in the output linesshould be equal to the number of bins in the correspondingworkspace. Conversely, if you use plot_bin, the number of elements inthe output lines should be equal to the number of spectra in theworkspace.
To modify the figure, you first need to obtain the figure objectthat represents the figure where the lines are displayed. Once you doso you can for example set the title of the figure like this:
Other properties can be modified using different functions, as inmatplotlib’s pyplot. For example:
By default, these functions manipulate the current figure (the last ormost recently shown figure). You can also save the current figure intoa file like this:
where the file format is guessed from the file extension. The sameextensions as in the MantidPlot figure export dialog are supported,including jpg, png, tif, ps, and svg.
The usage of these functions very similar to the matlab and/orpyplot functions with the same names. The list of functionscurrently supported is provided further below.
Additional options supported as keyword arguments (kwargs):¶
There is a couple of important plot options that are set as keywordarguments:
Option name | Values supported |
---|---|
error_bars | True, False (default) |
hold | on, off |
error_bars has the same meaning as in the traditional mantidplot plotfunctions: it defines whether error bars should be added to theplots. hold has the same behavior as in matplotlib and pyplot. If thevalue of hold is ‘on’ in a plot command, the new plot will be drawn ontop of the current plot window, without clearing it. This makes itpossible to make plots incrementally.
For example, one can add two spectra from a workspace using thefollowing command:
But similar results can be obtained by plotting one of the spectra bya first command, and then plotting the second spectra in a subsequentcommand with the hold parameter enabled:
After the two commands above, any subsequent plot command that passeshold=’on’ as a parameter would add new spectra into the same plot. Analternative way of doing this is explained next. Note however thatusing the hold property to combine different types of plots(plot_spectrum, plot_bin, etc.) will most likely produce uselessresults.
Multi-plot commands¶
In this version of pyplot there is limited support for multi-plotcommands (as in pyplot and matlab). For example, you can type commandslike the following:
This command will plot spectra 100 and 101 in red and spectra 200 and201 in blue on the same figure. You can also combine differentworkspaces, for example:
Style options supported as keyword arguments¶
Python Plot Figure Size
Unless otherwise stated, these options are in principle supported inall the plot variants. These options have the same (or as closed aspossible) meaning as in matplotlib.
Option name | Values supported |
---|---|
linewidth | real values |
linestyle | ‘-‘, ‘–’, ‘-.’ ‘.’ |
marker | None/”None” ‘o’, ‘v’, ‘^’, ‘<’, ‘>’, ‘s’, ‘*’,‘h’, ‘ ’, ‘_’ |
color | color character or string (‘b’, ‘blue’, ‘g’, ‘green’,‘k’, ‘black’, ‘y’, ‘yellow’, ‘c’, ‘cyan’, ‘r’, ‘red’.‘m’, ‘magenta’, etc.). RGB colors are not supported atthe moment. |
Modifying the plot axes¶
You can modify different properties of the plot axes via functions, asseen before. This includes the x and y axis titles, limits and scale(linear or logarithmic). For example:
An alternative is to use equivalent methods provided by the Figure andAxes objects. For this you first need to retrieve the figure and axeswhere a plot (or line) has been shown.
Functions that modify plot properties¶
Here is a list of the functions supported at the moment. They offerthe same functionality as their counterparts in matplotlib’spyplot.
- title
- xlabel
- ylabel
- ylim
- xlim
- axis
- xscale
- yscale
- grid
- savefig
3 Plots In One Figure Python Geeks
This is a limited list of functions that should be sufficient forbasic plots. These functions are presently provided as an example ofthis type of interface, and some of them provide functionality similaror equivalent to several of the keyword arguments for plot commandsdetailed in this documentation. Some others produce results equivalentto the more object oriented methods described above. For example, thefunction xlabel is equivalent to the method set_xlabel applied on theAxes object for the current figure.
This module is by default imported into the standard MantidPlotnamespace. You can use the functions and classes included here withoutany prefix or adding this module name prefix (pymantidplot.pyplot), asin the following example:
Note that the plot() function of this module has replaced thetraditional plot() function of MantidPlot which has been moved into apackage called qtiplot. To use it you can do as follows:
Below is the reference documentation of the classes and functionsincluded in this module.
A very minimal replica of matplotlib.axes.Axes. The true Axes is asublcass of matplotlib.artist and provides tons of functionality.At the moment this just provides a few set methods for propertiessuch as labels and axis limits.
- __init__(fig, xscale='linear', yscale='linear')¶
Set the boundaries or limits of the x and y axes
@param lims :: list or vector specifying min x, max x, min y, max y
Get the figure where this Axes object is included
Returns :: figure object for the figure that contains this Axes
Set the label or title of the x axis
@param lbl :: x axis lbl
Set the boundaries of the x axis
@param xmin :: minimum value@param xmax :: maximum value
Set the type of scale of the x axis
@param scale_str :: either ‘linear’ for linear scale or ‘log’ for logarithmic scale
Set the label or title of the y axis
@param lbl :: y axis lbl
Set the boundaries of the y axis
@param ymin :: minimum value@param ymax :: maximum value
Set the type of scale of the y axis
@param scale_str :: either ‘linear’ for linear scale or ‘log’ for logarithmic scale
A very minimal replica of matplotlib.figure.Figure. This class ishere to support manipulation of multiple figures from the commandline.
- __init__(num)¶
Obtain the list of axes in this figure.
- Returns :: list of axes. Presently only one Axes object is supported
- and this method returns a single object list
Helper method, returns the current sequence number for figures
Save current plot into a file. The format is guessed from the file extension (.eps, .png, .jpg, etc.)
@param name :: file name
Set a title for the figure
@param title :: title string
A very minimal replica of matplotlib.Line.Line2D. The true Line2Dis a sublcass of matplotlib.artist and provides tons offunctionality. At the moment this just provides get_xdata(),get_ydata(), and figure() methods. It also holds its Graphobject and through it it would be possible to provideadditional selected functionality. Keep in mind that providingGUI line/plot manipulation functionality would require a proxyfor this class.
- __init__(graph, index, x_data, y_data, fig=None)¶

Set the boundaries or limits of the x and y axes
@param lims :: list or vector specifying min x, max x, min y, max y
Return Figure object for a new figure or an existing one (if there is anywith the number passed as parameter).
@param num :: figure number (optional). If empty, a new figure is created.
Enable a grid on the active plot (horizontal and vertical)
@param title :: ‘on’ to enable
Plot the data in various forms depending on what arguments are passed. Currently supportedinputs: arrays (as Python lists or numpy arrays) and workspaces (by name or workspace objects).
@param args :: curve data and options@param kwargs :: plot line options
Returns :: the list of curves included in the plot
args can take different forms depending on what you plot. You can plot:
- a python list or array (x) for example like this: plot(x)
- a workspace (ws) for example like this: plot(ws, [100,101]) # this will plot spectra 100 and 101
- a list of workspaces (ws, ws2, ws3, etc.) for example like this: plot([ws, ws2, ws3], [100,101])
- workspaces identified by their names: plot([‘HRP39182’, ‘MAR11060.nxs’], [100,101])
You can also pass matplotlib/pyplot style strings as arguments, for example: plot(x, ‘-.’)
As keyword arguments (kwargs) you can specify multipleparameters, for example: linewidth, linestyle, marker, color.
An important keyword argument is tool. At the moment thefollowing values are supported (they have long and short aliases):
- To plot spectra: ‘plot_spectrum’ OR ‘spectrum’ OR ‘plot_sp’ OR ‘sp’ (default for workspaces).
- To plot bins: ‘plot_bin’ OR ‘bin’
- To do an MD plot: ‘plot_md’ OR ‘md’
Multiple Plots In Python
Please see the documentation of this module (use help()) for more details.
X-Y plot of the bin counts in a workspace.
Plots one or more bin, selected by indices, using spectra numbers as x-axis and bin counts for each spectrum as y-axis.
Python Plot New Figure
@param workspaces :: workspace or list of workspaces (both workspace objects and names accepted)@param indices :: indices of the bin(s) to plot
Returns :: the list of curves included in the plot
X-Y plot of an MDWorkspace.
@param workspaces :: workspace or list of workspaces (both workspace objects and names accepted)
Returns :: the list of curves included in the plot
X-Y Plot of spectra in a workspace.
Plots one or more spectra, selected by indices, using bin boundaries as x-axisand the spectra values in each bin as y-axis.
@param workspaces :: workspace or list of workspaces (both workspace objects and names accepted)@param indices :: indices of the spectra to plot, given as a single integer or a list of integers
Returns :: the list of curves included in the plot
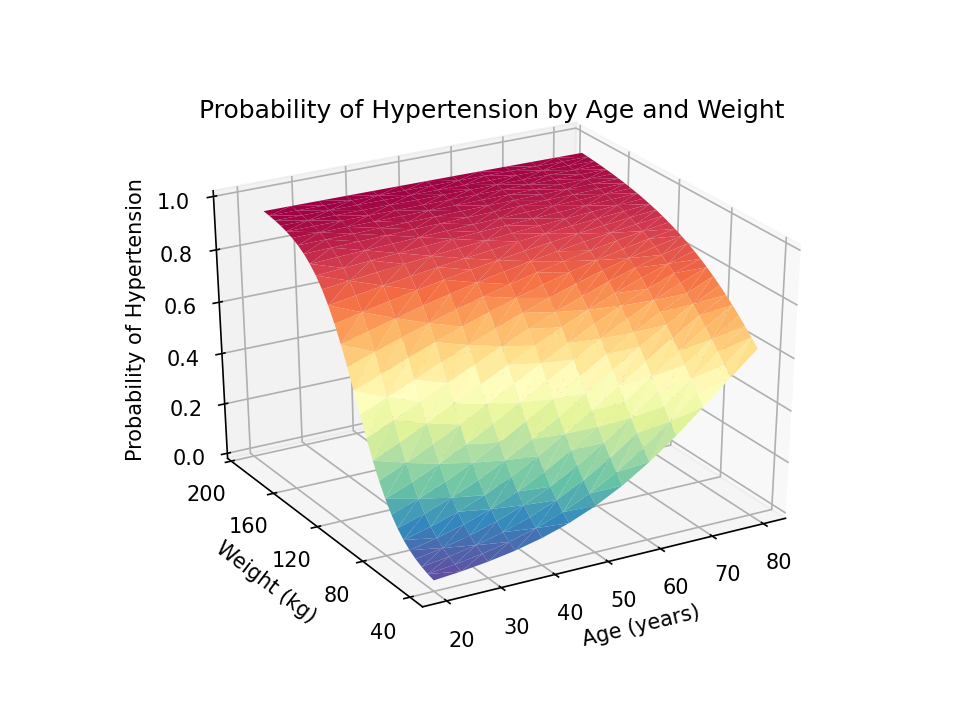
Save current plot into a file. The format is guessed from the file extension (.eps, .png, .jpg, etc.)
@param name :: file name
Set title of the active plot
@param title :: title string
Set the label or title of the x axis
@param lbl :: x axis lbl
Set the boundaries of the x axis
@param xmin :: minimum value@param xmax :: maximum value
Set the type of scale of the x axis
@param scale_str :: either ‘linear’ for linear scale or ‘log’ for logarithmic scale
Set the label or title of the y axis
@param lbl :: y axis lbl
Set the boundaries of the y axis
@param ymin :: minimum value@param ymax :: maximum value
Set the type of scale of the y axis
@param scale_str :: either ‘linear’ for linear scale or ‘log’ for logarithmic scale